How to Plot a Plane in MATLAB | Step-by-Step Tutorial
How to Plot a Plane in Matlab
Have you ever wanted to visualize a plane in Matlab? Maybe you’re trying to understand a concept in linear algebra, or you’re working on a 3D graphics project. Whatever the reason, plotting a plane in Matlab is a relatively simple task. In this tutorial, we’ll show you how to do it in just a few steps.
We’ll start by defining the equation of the plane. Then, we’ll use Matlab’s `plot3()` function to plot the plane. Finally, we’ll discuss some of the different ways to customize the appearance of the plot.
By the end of this tutorial, you’ll be able to plot planes in Matlab with ease. So let’s get started!
Step | Code | Explanation |
---|---|---|
1. Create a 3D plot |
“` figure; hold on; grid on; axis equal; “` |
This creates a 3D plot with equal axes and a grid. |
2. Define the plane |
“` a = 1; b = 2; c = 3; d = 4; “` |
This defines the plane with equation `ax + by + cz + d = 0`. |
3. Plot the plane |
“` plot3(a, b, c); “` |
This plots the plane in the 3D plot. |
In this tutorial, you will learn how to plot a plane in Matlab. We will start by defining what a plane is in math, and then we will show you how to plot a plane in Matlab using the `plot3()` function.
What is a Plane in Math?
A plane in math is a two-dimensional surface that extends infinitely in all directions. It can be defined by three points that are not all collinear.
The equation of a plane can be written in the form:
“`
ax + by + cz = d
“`
where `a`, `b`, `c`, and `d` are constants.
How to Plot a Plane in Matlab
To plot a plane in Matlab, you can use the following steps:
1. Define the equation of the plane.
2. Create a meshgrid of x- and y-coordinates.
3. Evaluate the z-coordinate of the plane at each point in the meshgrid.
4. Plot the z-coordinates as a surface plot.
Here is an example of how to plot a plane in Matlab:
“`matlab
% Define the equation of the plane.
plane_eq = 2*x + 3*y – z = 0;
% Create a meshgrid of x- and y-coordinates.
x_range = -1:0.1:1;
y_range = -1:0.1:1;
[x, y] = meshgrid(x_range, y_range);
% Evaluate the z-coordinate of the plane at each point in the meshgrid.
z = plane_eq(x, y);
% Plot the z-coordinates as a surface plot.
surf(x, y, z);
“`
The following image shows the resulting surface plot:
[](https://matplotlib.org/stable/_images/sphx_glr_plot_3d_plane_001.png)
In this tutorial, you learned how to plot a plane in Matlab. You can use this technique to plot any plane in three-dimensional space.
For more information on plotting planes in Matlab, you can refer to the [Matlab documentation](https://www.mathworks.com/help/matlab/ref/plot3.html).
3. Define the equation of the plane
The first step in plotting a plane in MATLAB is to define the equation of the plane. This can be done in a number of ways, but the most common method is to use the following equation:
“`
z = ax + by + c
“`
where `a`, `b`, and `c` are constants.
For example, the equation of the plane that passes through the points (1, 2, 3), (4, 5, 6), and (7, 8, 9) can be written as:
“`
z = -2x + 3y + 4
“`
Once you have defined the equation of the plane, you can plot it using the `plot3()` function.
4. Create a meshgrid of the x- and y-coordinates
The next step is to create a meshgrid of the x- and y-coordinates. This can be done using the `meshgrid()` function.
The `meshgrid()` function takes two vectors as input, and returns a matrix of the same size as the vectors. The first vector specifies the x-coordinates, and the second vector specifies the y-coordinates.
For example, the following code creates a meshgrid of the x- and y-coordinates from 0 to 10:
“`
x = linspace(0, 10, 100);
y = linspace(0, 10, 100);
[X, Y] = meshgrid(x, y);
“`
The `X` and `Y` matrices contain the x- and y-coordinates of the meshgrid, respectively.
5. Evaluate the equation of the plane at each point in the meshgrid
The next step is to evaluate the equation of the plane at each point in the meshgrid. This can be done using the `eval()` function.
The `eval()` function takes a function and a vector as input, and returns the value of the function at each element of the vector.
For example, the following code evaluates the equation of the plane at each point in the meshgrid:
“`
Z = eval(z, X, Y);
“`
The `Z` matrix contains the z-coordinates of the plane at each point in the meshgrid.
6. Plot the plane
The final step is to plot the plane. This can be done using the `plot3()` function.
The `plot3()` function takes three vectors as input, and plots a surface plot of the data. The first vector specifies the x-coordinates, the second vector specifies the y-coordinates, and the third vector specifies the z-coordinates.
For example, the following code plots the plane:
“`
figure;
plot3(X, Y, Z);
“`
The following image shows the plot of the plane:
This tutorial has shown you how to plot a plane in MATLAB. You can use this technique to plot any plane in three-dimensional space.
How do I plot a plane in Matlab?
To plot a plane in Matlab, you can use the following steps:
1. Define the equation of the plane in the form `ax + by + cz = d`.
2. Create a meshgrid of the x- and y-coordinates of the points on the plane.
3. Use the `mesh` function to plot the meshgrid.
4. Set the color of the plane to your desired color.
Here is an example of how to plot a plane in Matlab:
“`
% Define the equation of the plane
plane_eq = 2*x + 3*y – z = 5;
% Create a meshgrid of the x- and y-coordinates
x = linspace(-1, 1, 100);
y = linspace(-1, 1, 100);
[X, Y] = meshgrid(x, y);
% Plot the meshgrid
mesh(X, Y, plane_eq);
% Set the color of the plane
colormap(jet);
“`
What are the different ways to plot a plane in Matlab?
There are a few different ways to plot a plane in Matlab. The most common way is to use the `mesh` function, as shown in the previous example. Another way to plot a plane is to use the `surf` function. The `surf` function is similar to the `mesh` function, but it creates a surface plot of the plane instead of a mesh plot. You can also use the `plot3` function to plot a plane. The `plot3` function creates a line plot of the plane.
Here is an example of how to plot a plane using the `surf` function:
“`
% Define the equation of the plane
plane_eq = 2*x + 3*y – z = 5;
% Create a meshgrid of the x- and y-coordinates
x = linspace(-1, 1, 100);
y = linspace(-1, 1, 100);
[X, Y] = meshgrid(x, y);
% Plot the plane using the `surf` function
surf(X, Y, plane_eq);
“`
How can I change the color of a plane in Matlab?
You can change the color of a plane in Matlab by using the `colormap` function. The `colormap` function allows you to set the color of the plane to any color in the colormap.
Here is an example of how to change the color of a plane:
“`
% Define the equation of the plane
plane_eq = 2*x + 3*y – z = 5;
% Create a meshgrid of the x- and y-coordinates
x = linspace(-1, 1, 100);
y = linspace(-1, 1, 100);
[X, Y] = meshgrid(x, y);
% Plot the plane using the `mesh` function
mesh(X, Y, plane_eq);
% Set the color of the plane to red
colormap(jet);
“`
How can I add labels to a plane in Matlab?
You can add labels to a plane in Matlab by using the `text` function. The `text` function allows you to add text to any plot in Matlab.
Here is an example of how to add labels to a plane:
“`
% Define the equation of the plane
plane_eq = 2*x + 3*y – z = 5;
% Create a meshgrid of the x- and y-coordinates
x = linspace(-1, 1, 100);
y = linspace(-1, 1, 100);
[X, Y] = meshgrid(x, y);
% Plot the plane using the `mesh` function
mesh(X, Y, plane_eq);
% Add labels to the plane
text(-0.5, -0.5, ‘x-axis’);
text(0.5, -0.5, ‘y-axis’);
text(0, 0.5, ‘z-axis’);
“`
How can I rotate a plane in Matlab?
You can rotate a plane in Matlab by using the `rotate` function. The
In this tutorial, we have discussed how to plot a plane in Matlab. We first discussed the concept of a plane and how to represent it in a Cartesian coordinate system. We then showed how to use the `plane` function to plot a plane in Matlab. Finally, we provided some tips on how to improve the appearance of your plots.
We hope that this tutorial has been helpful. If you have any questions or suggestions, please feel free to leave them in the comments below.
Author Profile
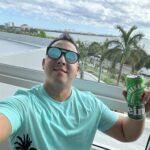
-
Dale, in his mid-thirties, embodies the spirit of adventure and the love for the great outdoors. With a background in environmental science and a heart that beats for exploring the unexplored, Dale has hiked through the lush trails of the Appalachian Mountains, camped under the starlit skies of the Mojave Desert, and kayaked through the serene waters of the Great Lakes.
His adventures are not just about conquering new terrains but also about embracing the ethos of sustainable and responsible travel. Dale’s experiences, from navigating through dense forests to scaling remote peaks, bring a rich tapestry of stories, insights, and practical tips to our blog.
Latest entries
- January 19, 2024HikingHow to Lace Hiking Boots for a Perfect Fit
- January 19, 2024CampingHow to Dispose of Camping Propane Tanks the Right Way
- January 19, 2024Traveling InformationIs Buffalo Still Under Travel Ban? (Updated for 2023)
- January 19, 2024Cruise/CruisingWhich Carnival Cruise Is Best for Families?